Nest Js, with its powerful and intuitive features, is rapidly becoming the go-to framework for JavaScript developers around the globe. Among these features, error handling plays a crucial role in developing resilient applications that ensure a seamless user experience. Thorough knowledge of how to deal with errors in Nest Js is paramount to creating high-quality, high-performing applications. This document provides an in-depth exploration of error handling in Nest Js, from basic concepts to advanced techniques, aiming to equip enthusiasts and hobbyists with the skills and knowledge needed to excel in this area.
Understanding Errors in Nest Js
Understanding Errors in Nest Js
Errors in Nest Js, as in any software or application framework, signify an abnormal or unexpected event or condition encountered by the program during runtime. These could range from type errors and reference errors to syntax and logical errors. The occurrence of such errors is generally a signal that something went wrong somewhere in the code, which prevented normal execution from taking place. Errors in Nest Js need to be identified, isolated, and handled effectively to ensure uninterrupted functionality of the application.
Understanding Exceptions in Nest Js
In the context of Nest Js, exceptions are special instances of errors that indicate significant deviations from expected application behaviour. Generally unexpected, these exceptions halt the program execution when encountered during runtime unless manageably handled. Nest Js provides an Exceptions layer that allows for clean, uncomplicated exception handling, following the principle of separation of concerns.
HttpException and Its Significance
The HttpException is a built-in utility that Nest Js offers to handle HTTP-based exceptions. It enables the configuration of an HTTP response explicitly, allowing the developer to control the status code, response headers, and response body. Generating an HttpException is easy, simply needing to throw an instance of the HttpException object, with the response string or object and status code as arguments.
Error Handling Filters
Error filters in Nest Js provide a robust mechanism to handle and process exceptions. Every exception thrown by a route handler, whether an HttpException or not, passes through a series of exception filters that users define. These filters give developers granular control over error handling, exceptions, and response formatting.
Using Custom Exceptions
Nest Js allows developers to define custom exceptions by extending the base HttpException class. This custom exception can encapsulate specific logic that characterizes an unusual situation in an application-specific domain. Including a necessary status code, response object or string, the developer can have an increased level of control over exception handling.
Unhandled Exceptions
Unhandled exceptions are those errors or exceptions that the system generates but do not handle within your application. These types of exceptions, if not addressed, can crash the application or lead to unspecified behavior. Handling these exceptions is crucial to maintain the system’s stability and reliability. Nest Js provides several tools and options to capture and address unhandled exceptions, such as global filters and pipes.
Understanding Exception Filters
Exception filters in Nest Js provide a mechanism to catch thrown exceptions and format responses. Exception filters can be bound on many levels – globally, per-module, per-controller, or even per individual route handlers, giving developers a great deal of flexibility.
Grasping the concept of handling errors and exceptions effectively in Nest Js is paramount. This not only empowers you to build robust and reliable applications, but it also enhances maintainability. The ability to swiftly respond to, and resolve unexpected system behaviors, is a core skill in software development.
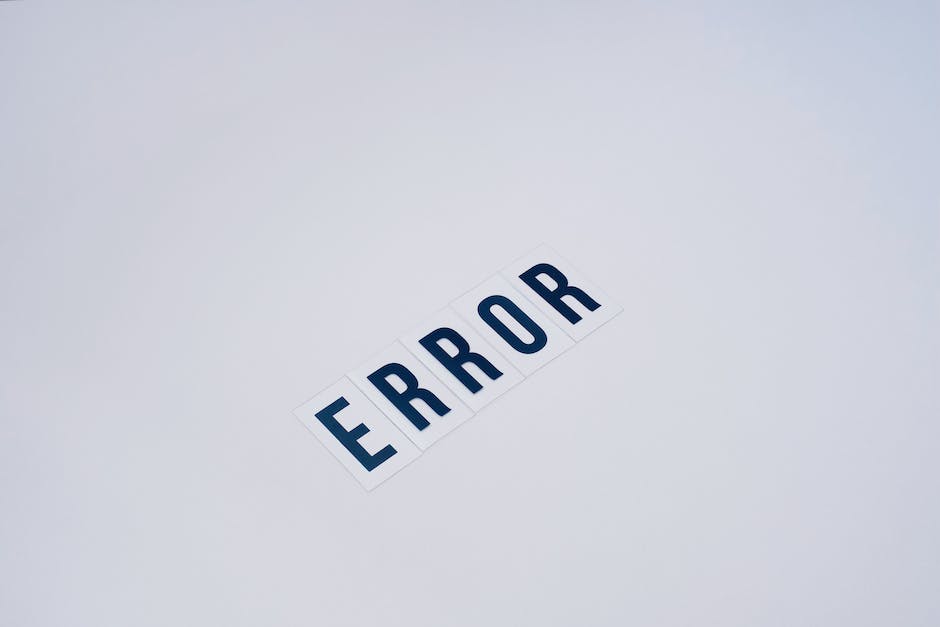
Exception Filters in Nest Js
Diving Deep into Exception Filters
In the world of Nest Js, one cannot underestimate the importance of Exception Filters. These crucial tools help you as a developer to manage error handling processes in a streamlined manner. They cleverly intercept any exception tossed out from middleware, route handlers, or even other exception filters, enabling the implementation of a response sequence you have defined. This makes your code not only efficient but also predictable in the face of unexpected scenarios.
Creating an Exception Filter
To create an exception filter, you need to define a class and decorate it with the @Catch()
decorator, which comes from the @nestjs/common
package. This decorator takes the Exception class the filter should catch, or a list of Exceptions when catching multiple types. The class must then implement the ExceptionFilter
interface and the single catch()
method it requires, which takes two parameters: the exception and an ArgumentHost object.
Implementing Built-In Exception Filters
Nest Js comes with built-in Exception filters for standard HTTP responses, these include BadRequestException
, UnauthorizedException
, and NotFoundException
among others. These Exceptions can be thrown from route handlers using the @nestjs/common
package and Nest Js will automatically send the appropriate HTTP response and status code.
For instance, throwing a NotFoundException
will result in a 404 response with the message of the Exception.
These built-in Exception filters can be extended and customized to cater to specific needs in an application. Custom messages can be passed to the constructor and additional parameters can be included to extend the capabilities of these filters beyond the default implementation.
Binding Exception Filters
Exception filters can be bound at different levels in a Nest Js application. They can be bound at the method scope level, controller scope level, or the global level, depending on the specific requirements of the application.
To bind a filter to a specific method or controller, the @UseFilters
decorator can be used. This decorator takes a filter class or an instance of the filter class as input. This level of binding ensures that the filter captures only Exceptions thrown from within the decorated context.
For global-level binding, Exception filters can be bound in a main file or within a module by using the app.useGlobalFilters()
method. This captures all Exceptions thrown from any part of the application and applies the filter to them.
Exception filters in Nest Js are an invaluable tool in creating applications with effective and efficient error handling. These filters allow you to create customized responses to specific exceptions and bind them according to the needs of your application. Acquiring knowledge and proficiency in Exception filters can bolster the robustness of your Nest Js projects.
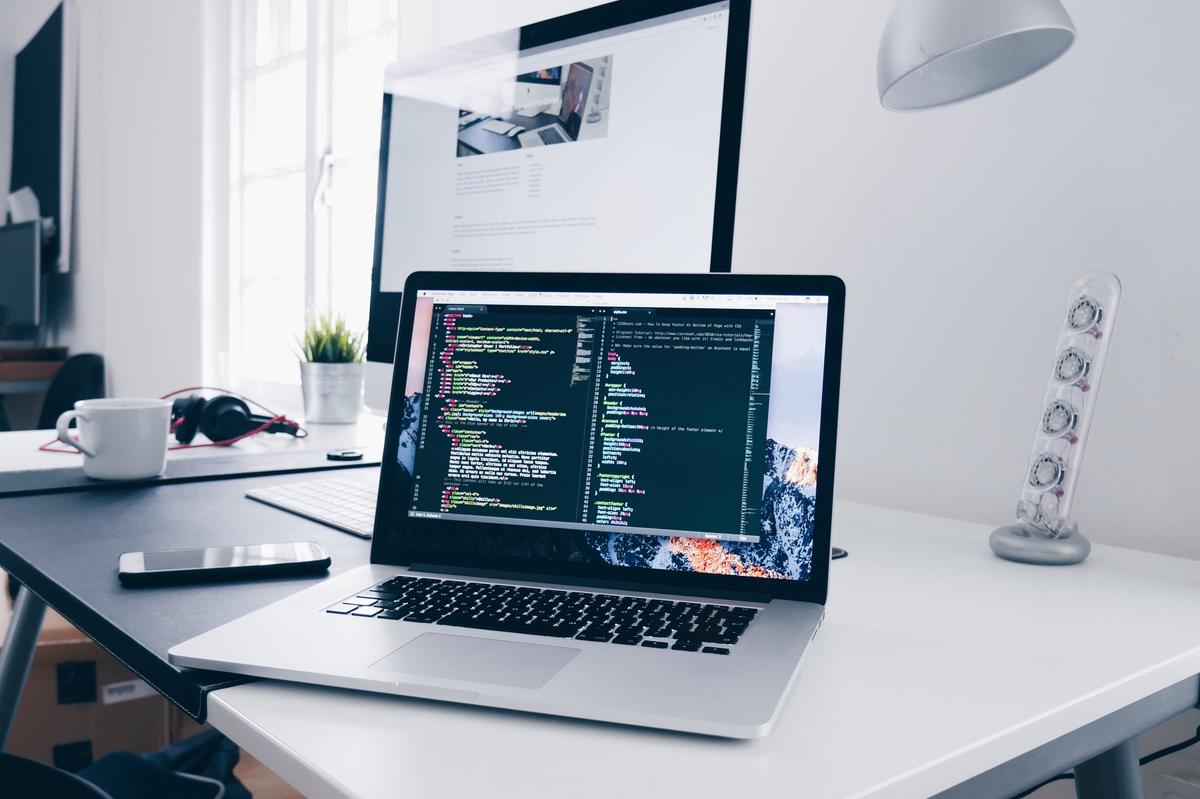
Using Interceptors for Error Handling
Diving Deeper: The Role of Interceptors in Nest Js
The topic of error handling in Nest Js doesn’t end with Exception Filters; it continues with another critical component – Interceptors. These tools provide an organized structure to manage and handle errors, enveloping and controlling both incoming HTTP requests and outgoing HTTP responses. This level of control improves error management and increases the maintainability of your codebase.
Interceptors in Nest Js are centered around ‘pointcuts’ – designated trigger points in the code that activate the interceptor. Once activated, these Interceptors examine and manage the data exchange, perform needed modifications, and then propagate the data further. Think of Interceptors as a diligent middleman, making essential interventions to ensure a smooth and error-free data flow.
Transforming Error Responses with Interceptors
One of the breakthrough perks of Interceptor utilization in Nest Js is their ability to transform error responses into desired formats. This is particularly beneficial when designing API responses, as it enables a consistent output regardless of any underlying service flaws or errors.
In practice, Interceptors allow you to catch errors before they reach the end-user, mold the response into a format that aligns with the application’s protocols, and then transfer the transformed error data properly. This conversion provides a far more user-friendly and comprehensible error message that aids in understanding and rectifying issues.
The Exception Handler for Enhanced Error Response Control
To gain even greater error response control, developers often turn to the Exception handler in Nest Js. This class assists in catching exceptions and formatting suitable error responses while allowing the continuation of application execution. It empowers developers to tailor their error messages, providing more clarity and direction to rectify issues.
The Exception handler can catch exceptions from various sources including HTTP requests, WebSockets, Microservices, GraphQL, and more, offering comprehensive error management in any application context.
The Transform Decorator in Error Management
The Transform decorator is another powerful tool in error management within Nest Js. By using this decorator, developers can manipulate incoming or outgoing data in various ways. This becomes particularly handy while modifying error responses or exceptions to align with the desired format of the application.
When errors pop up, they can be intercepted, processed through the Transform decorator, and converted into a more manageable and understandable format. The output could range from a simpler error message to an elaborate exception response, all based on the developer’s discretion.
An Overview of Error Handling in Nest Js
For enthusiasts like you who are aiming to hone their skills in error handling, Nest Js offers a comprehensive framework enriched with robust classes and decorators. The strategic use of these resources can enhance error management and responses, bolstering the sturdiness, upkeep, and usability of your applications. As you venture into mastering this aspect of Nest Js, you’ll find that it significantly streamlines the process of developing and maintaining high-quality software.
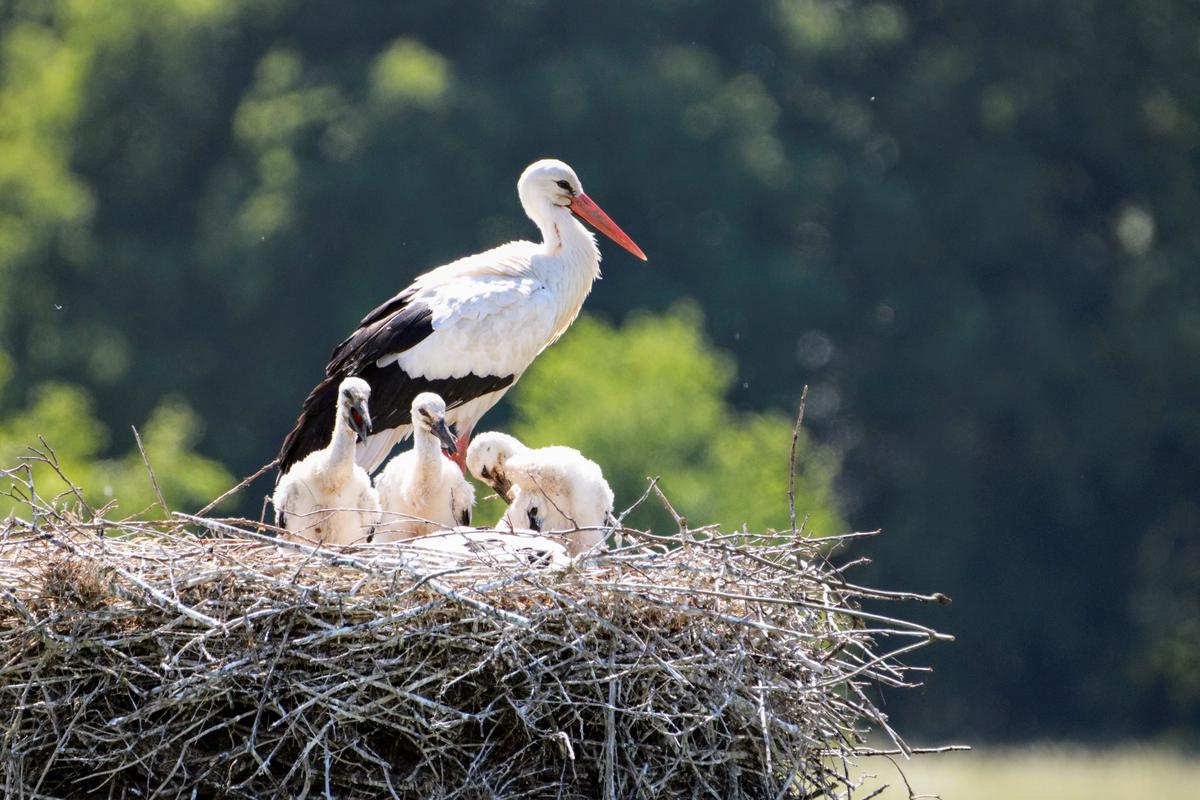
Photo by mschalker on Unsplash
Implementing Pipes for Validation and Error Handling
Diving Into Pipes in Nest Js
Transitioning from the overall error handling framework, it’s crucial to gain an understanding of one of its key components – pipes. Existing right in the heart of the middleware and sprung into action just before the route handler, pipes offer you a range of control over incoming data – you can validate it, manipulate it and so much more. From data transformations and sanitization to pre-computation, pipes wear many hats and handle vital responsibilities. The data they refine and return eventually find their way to the route handlers in the controller.
Another important aspect to keep in mind is that pipes can throw exceptions. When this happens, it triggers the error handlers in Nest Js, bringing into play the power of its sophisticated error handling mechanisms. This function of pipes shields your route handlers from dealing with inappropriate data and consequently boosts the security of your application.
Building a Custom Pipe
Building a custom pipe to cater to your specific needs involves creating a new class implementing the PipeTransform interface provided by Nest Js, and implementing a ‘transform()’ method where the logic goes.
This class can be structured to conduct rigorous checks and validation of the incoming data. If any abnormalities or errors are detected, exceptions can be thrown, transferring control to Nest Js’s global error handlers. The ‘transform()’ method should finally return the valid data, ready to be used by the route handler.
Using Built-In ValidationPipe
Nest Js also offers a built-in ValidationPipe that you can utilize for basic validations. The ValidationPipe leverages class-validator and class-transformer packages to validate incoming data based on some predefined rules and decorators.
If the data is invalid or does not meet the stipulated validation criteria, the ValidationPipe will automatically throw an HTTP exception with appropriate status and a message detailing the validation errors. This simplified mechanism saves you time and energy, and adds an additional layer of robust protection against invalid incoming data.
To make maximum use of this mechanism, always apply the ValidationPipe as a global pipe at the application level, ensuring that all incoming requests pass through it.
Enhancing Error Handling with Filters
While pipes allow you to reject invalid data early in the request handling pipeline, Nest Js also provides built-in exception filters to allow for application-wide error handling.
Exception filters are specific classes endowed with a ‘catch()’ method to intercept unhandled exceptions across your whole application or a subset of your routes. These filters can help you standardize error responses, send alerts if an error occurs, or even attempt a corrective action in response to a particular exception.
You can either use built-in exception filters or create custom filters to serve your specific needs and preferences, ensuring that you not only throw exceptions, but also handle them in the most efficient manner.
Leveraging Interceptors for Error Responses
In addition to pipes and filters, Nest Js offers interceptors to handle the return leg of the processing cycle. Interceptors have a ‘handle()’ method which wraps around the route handler for a given endpoint, allowing you to manipulate the request processing pipeline both before and after the route handler is invoked.
Interceptors can glean meaningful insights from thrown errors, such as the frequency of occurrence or the specific part of the codebase where they occur most, and facilitate more descriptive error reports and responses. This can greatly enhance your error handling and debugging approaches.
Summarily, the employment of pipes, filters, and interceptors in Nest Js offers not only the ability to validate incoming data and preemptively detect errors, but also provides dynamic methods to manage those errors. This can greatly enhance the robustness and resilience of your application.
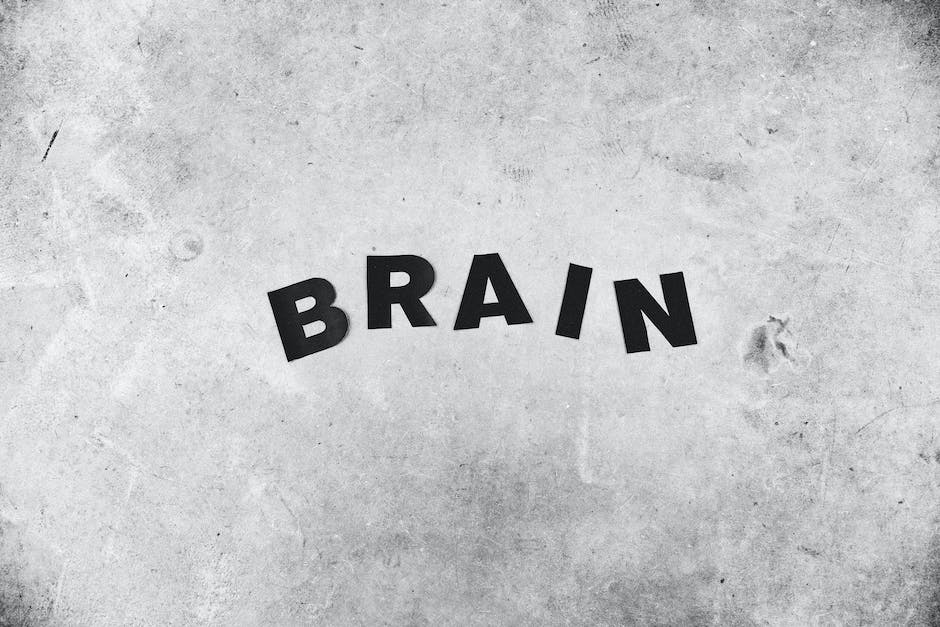
Advanced Error Handling Techniques
Tackling the Challenge of Error Handling in Nest Js
To achieve a sturdy and reliable application, the efficient management of errors and exceptions is fundamental. In Nest Js, any unhandled exception is directed to an exception filter, a key component within the Exception Filters layer.
Understanding Exception Filters Layer
Exception filters in Nest Js catch unhandled exceptions thrown from within your route handlers (middleware also) and provide a centralized place for exception handling. They return a user-friendly response, log the exceptions to your database, or send them to an external service for further analysis.
If you want to override the default behavior, you must create a filter using the @Catch()
decorator. The decorator binds the metadata of the exceptions being caught to the exception filter itself.
Custom HTTP Error Responses
To customize the HTTP error response that is sent when an exception is not handled, you can create custom exceptions that extend the HttpException
class and provide the HTTP response internally. This ensures more granular error handling and better user feedback.
Using Third-Party Libraries
Libraries such as ‘express-async-errors’ allow you to handle exceptions asynchronously in a more robust way than traditional try-catch blocks. For example, you can use this library to create global error middleware that catches and handles any uncaught exceptions in your Express application.
Interceptors for Transforming Error Responses
In Nest Js, interceptors can also be used in error handling. They’re used to bind extra logic before or after method execution. Moreover, they can transform the result returned from a function or handle errors thrown inside.
Simply create a custom interceptor and, in its catch()
method, find and transform specific exceptions, allowing you to determine how these exceptions will appear once they’ve been thrown.
Exception Handling Best Practices
Thoroughly cover every part of your code with try-catch blocks. However, avoid cluttering your code base by focusing on parts of your code that actually have a chance of throwing an error. If possible, create custom exceptions which can offer more clarity about the errors.
Prioritize logging every error in your system, this will save you countless hours when trying to debug errors. Make sure your messages are clear and give enough context to understand the problem.
You might leverage the benefits of logging services like Loggly, Papertrail, or Sentry that offer advanced features such as log aggregation, searching, or error alerting.
Remember, robust error handling makes your application more predictable and easy to debug, as any error that might occur will be caught and properly handled, providing feedback on what went wrong, and maybe how it can be fixed.
Photo by markkoenig on Unsplash
Mastering error handling in Nest Js opens doors to an improved coding environment where bugs and glitches are addressed proactively. A strong grasp of Exception Filters, Interceptors, and Pipes, as well as advanced techniques and tools provides the necessary tools to maintain optimal application performance and usability. Embracing the principles and practices presented in this resource will surely lead to the development of robust, reliable, and high-performing applications. Adopt these strategies and techniques to your Nest Js journey and join the leagues of developers who are shaping the future of JavaScript development.
Writio: Transforming content creation, the AI content writer that crafts captivating articles. This post was written by Writio.