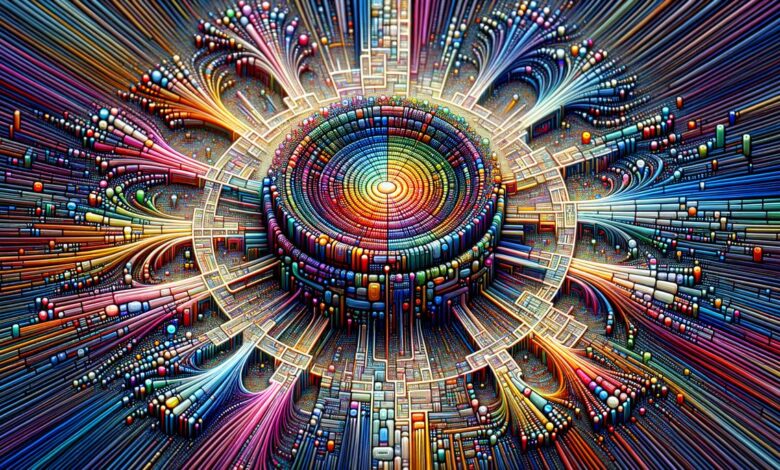
Autoencoders are neural networks that excel in unsupervised learning, particularly in data compression and noise reduction. They convert inputs into low-dimensional representations and then reconstruct the original data, offering a powerful approach to unsupervised learning. This article explores the architecture, types, training processes, and applications of autoencoders in deep learning.
What is an Autoencoder?
Autoencoders are neural networks that compress input data into a low-dimensional representation and then reconstruct it. The process involves three main components:
- Encoder: Compresses the input data, reducing its dimensionality while retaining critical features.
- Bottleneck: The most compact form of the compressed data, forcing the network to retain only essential information.
- Decoder: Reconstructs the original input from the low-dimensional representation.
Autoencoders are effective in tasks like data compression and noise reduction, balancing dimensionality reduction with accurate data reconstruction. Research has shown that autoencoders can achieve compression ratios of up to 10:1 while maintaining high reconstruction quality1.
Architecture of Autoencoders
The autoencoder architecture consists of three key components working together:
- Encoder: Transforms high-dimensional data into a lower-dimensional format through multiple layers, retaining significant features while discarding redundant information.
- Bottleneck: The smallest layer in terms of dimensionality, ensuring only essential data features pass through. It limits information flow, compelling effective data compression and preventing overfitting.
- Decoder: Reverses the compression performed by the encoder, reconstructing the original input from the condensed data. It uses layers that gradually increase in size, mirroring the encoder's compression stages in reverse.
This architecture enables autoencoders to distill large amounts of data into crucial elements and reconstruct them efficiently, making them useful for tasks requiring dimensionality reduction, noise reduction, and data generation. The power of autoencoders lies in their ability to learn compact representations without explicit supervision.
Types of Autoencoders
- Undercomplete autoencoders: Feature a bottleneck layer with fewer dimensions than the input, forcing the network to learn the most important data features. They excel in dimensionality reduction tasks.
- Sparse autoencoders: Apply sparsity constraints during training, encouraging only a fraction of neurons to be active. This approach is useful for capturing diverse features in scenarios like anomaly detection.
- Contractive autoencoders: Focus on making learned representations robust to small input changes by adding a penalty to the loss function. They're suitable for tasks requiring stability in feature extraction.
- Denoising autoencoders: Take corrupted data as input and learn to reconstruct the original, clean version. They're valuable for image and audio denoising tasks.
- Variational autoencoders (VAEs): Impose a probabilistic structure on the latent space, facilitating the generation of new, coherent data. They're useful in generative modeling tasks like creating new images or text.
Each type offers unique characteristics, allowing researchers to select the most suitable variant based on their specific application needs. For instance, VAEs have shown remarkable success in generating realistic human faces and handwritten digits2.
Training Autoencoders
Training autoencoders involves tuning several key hyperparameters:
- Code size (bottleneck size): Determines the degree of compression.
- Number of layers: Influences the model's capacity to capture complex patterns.
- Number of nodes per layer: Typically decreases in the encoder and increases in the decoder.
- Reconstruction loss function: Depends on data type and task requirements. Common choices include Mean Squared Error (MSE) for continuous data and Binary Cross-Entropy (BCE) for binary or normalized data.
The training process typically follows these steps:
- Initialize the model
- Compile the model with an appropriate optimizer and loss function
- Train the model by feeding input data and minimizing reconstruction loss
- Monitor performance using validation data to avoid overfitting
Fine-tuning these parameters is essential to achieve optimal performance for the specific application at hand. Careful selection of hyperparameters can significantly impact the autoencoder's ability to learn meaningful representations and generalize well to unseen data.
Applications of Autoencoders
Autoencoders have become useful tools in various practical applications across different domains. Their ability to condense information through encoding and then reconstruct the original data through decoding makes them versatile in handling tasks like:
- Dimensionality reduction
- Image denoising
- Data generation
- Anomaly detection
In dimensionality reduction, undercomplete autoencoders excel by reducing data without significant loss of information. This facilitates more efficient storage, faster computation, and effective data visualization. In genomics, autoencoders help compress high-dimensional data into manageable sizes while preserving critical genetic information. Similarly, in image processing, autoencoders can reduce the dimensions of high-resolution images, making tasks like image retrieval and clustering more computationally feasible.
Autoencoders are effective in image denoising. Denoising autoencoders are trained to remove noise from corrupted images by learning to reconstruct the original, clean images from noisy inputs. This is valuable in fields such as medical imaging, where clarity is vital. For example, in MRI or CT scans, denoising autoencoders can clean images, ensuring higher fidelity and better diagnostic accuracy.
Variational Autoencoders (VAEs) can generate new, realistic data samples similar to the original training data. This is achieved by treating the latent space as probabilistic, allowing for the creation of new data points through random sampling. In creative industries, VAEs can be used to generate new artworks or music. In research, they can help in simulating molecular structures in drug discovery.
In time-series data, autoencoders can generate realistic sequences based on historical data. This finds applications in stock market prediction and weather forecasting.
Anomaly detection is another area where autoencoders show utility. Trained to reconstruct data, autoencoders can identify anomalies by assessing reconstruction errors. This application is beneficial in:
- Cybersecurity
- Manufacturing
- Fraud detection in financial transactions
- Healthcare (analyzing electronic health records)
- Predictive maintenance (analyzing sensor data from industrial equipment)
These applications demonstrate the versatility of autoencoders in modern data-driven domains. From enhancing image quality and compressing data to detecting anomalies and generating new data, autoencoders play a role in harnessing the power of deep learning for practical solutions.
Advanced Techniques: JumpReLU SAE
JumpReLU SAE represents an advancement in sparse autoencoders, introducing a dynamic feature selection mechanism that improves performance and interpretability. Traditional sparse autoencoders enforce sparsity by maintaining a global threshold value for neuron activation, typically using ReLU functions. This method can be rigid, preserving irrelevant features with marginal activation values.
JumpReLU SAE addresses these limitations by implementing a novel activation function—dynamically determining separate threshold values for each neuron in the sparse feature vector. This approach enables the autoencoder to make more granular decisions about which features to activate, improving its ability to discern significant data attributes.
Key Features of JumpReLU SAE:
- Dynamic adjustment of activation thresholds based on specific data
- Optimization of thresholds during training
- Minimization of "dead features" (neurons that never activate)
- Mitigation of hyperactive neurons
- Enhanced interpretability of neural network activations
The core enhancement lies in JumpReLU's capacity to adjust activation thresholds based on the specific data being processed. During training, the network optimizes these thresholds, allowing neurons to become sensitive to distinct features. This mechanism bolsters the network's proficiency in compressing activations into a compact set of sparse features that are more efficient and aligned with human-readable concepts.
"JumpReLU SAE has demonstrated superior reconstruction fidelity compared to conventional SAE architectures. Across varied sparsity levels, it consistently delivers higher accuracy in reconstructing the original data while adhering to sparsity constraints."
One application is in the interpretability of large language models (LLMs), where understanding the representation of activations within the network is important. By applying JumpReLU SAE to large models, researchers can decompose complex activation patterns into smaller, more understandable components. This transparency is useful for tracing how LLMs generate language, make decisions, or respond to queries.
In summary, the JumpReLU SAE architecture enhances sparse autoencoders by introducing dynamic feature selection, addressing the limitations of static threshold methods. It ensures more effective and interpretable feature extraction, promoting a clearer understanding of neural network activations.
Autoencoders are useful for compressing and reconstructing data, making them valuable in various applications. Their versatility and effectiveness are notable in tasks such as dimensionality reduction, image denoising, and anomaly detection.
Writio: AI content writer for website publishers and blogs. This article was written by Writio.